Variable Names
-
variables user defined identifiers
-
variables are case sensitive, numVar and numvar are two different
variables
-
All variables must be declared before they are used
Standard IO
- C provides a set of functions in the header file stdio.h to read inputs and write outputs.
- C treats all devices as files:
Standard File | File Pointer | Device |
---|---|---|
Standard Input | stdin | Keyboard |
Standard Output | stdout | Screen |
Standard Error | stderr | your Screen |
Formatting Outputs - printf()
int printf (char *format , arg1 , arg2 , ...)
- printf prints a list of arbitrary arguments (arg1, arg2, ...) on the standard output under control of the format
- returns the number of characters printed
- format contains two types of objects:
- ordinary characters: printed to the output stream
- specifiers: causes conversion and printing of the next successive argument
Format Spceifiers
- %d (%i) int signed decimal
- %u int unsigned decimal
- %o int unsigned octal value
- %x (%X) int unsigned hex value
- %f float or double
- %e (%E) float or double exponential format
- %s array of char (string)
- %p pointer address stored in pointer
- %ld long signed decimal
- %hd short signed decimal
Reading inputs - scanf()
int scanf (char *format , arg1 , arg2 , ...)
- scanf reads characters from the keyboard, interprets them according to the specifiers in format, and stores the results through the remaining arguments
- each argument after format is a pointer (&c) indicates the memory address where the input should be store
Relational and Logical Operators
- Relational Operators: ==, !=, >, <, >=, <=
- Logical Operators: &&, ||, !
Bitwise Operators
- Bitwise Operators: &, |, ^, ⇠, <<, >>
- bit manipulation operators, only used with integer datatypes
Assignment Operators
- Assignment Operators: =, +=, -=, *=, /=, %=, &=, |=, ^=, <<=, >>=
- Most binary operators op have a corresponding assignment operator op=
Misc Operators
- Misc Operators: sizeof(), & (address of a variable), * (pointer to a variable), ?: (if?then:otherwise)
Pointer and Addresses (* and &)
The unary operator * is the indirection or dereferencing operator; when applied to a pointer, it accesses the object the pointer points to
int x =1, y =2;
int *ip; /* ip is a pointer to int */ ip =&x; /* ip now points to x */
y = *ip; /* y is now 1 */
*ip = 0; /* x is now 0 */
Type Conversion
- In arithmetic and bitwise operation the narrower operand will be converted to the wider one before applying the operation
- char -->short --> int --> unsigned int --> long --> unsigned long --> long long --> float --> double --> long double
- expressions like assignment might result in losing information like assigning longer integer to shorter or a float to an integer (may draw a warning)
Function
- every C program has at least one function: main()
- you can devide you program into sepatate functions, each function perform a specfic task
- The C standard library provides numerous built-in functions: printf() and scanf()
- The function declaration consists of:
- the function’s name
- return type
- list of parameters
- A function definition provides the actual body of the function
Static Variables
- the compiler to keeps the static variables in existence during the life-time of the program
- local variables static allows them to maintain their values between function calls
Memory Layout of C Programs
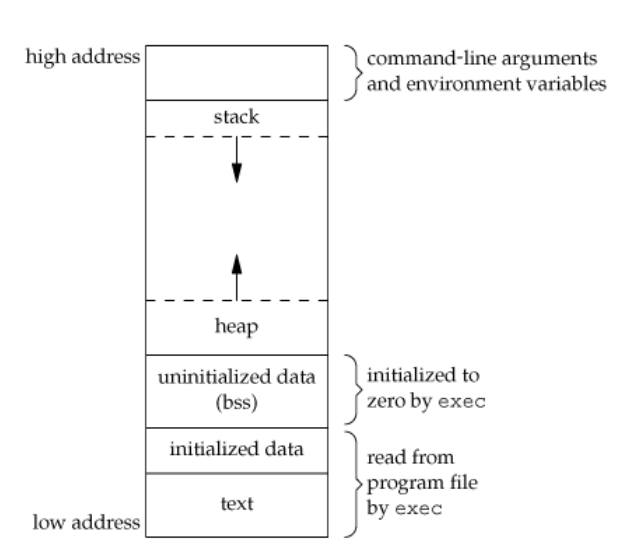
- A typical memory representation of C program consists of following sections
- Text segment
- Initialized data segment
- Uninitialized data segment
- Stack
- Heap
Text Segment
- text segment, also known as a code segment or text
- contains executable instructions
- may be placed below the heap or stack in order to prevent heaps and stack overflows from overwriting it
- shareable: single copy needs to be in memory for frequently executed programs
- readonly: to prevent a program from accidentally modifying its instructions
Initialized Data Segment
- simply the Data Segment: is a portion of virtual address space of a program,
- contains the global variables and static variables that are initialized by the programmer
- is not read-only since the values of variables can be altered at runtime
Uninitialized Data Segment
- often called the "bss" segment, "block started by symbol"
- Data in this segment is initialized by the kernel to arithmetic 0 before the program starts executing
- contains all global variables and static variables that are initialized to zero or do not have explicit initialization in source code, for instance:
Stack
- A "stack pointer" tracks the top of the stack
- The set of values pushed for one function call is termed a "stack frame"; A stack frame consists at minimum of a return address
- Each time a function is called, the address of where to return to and certain information about the caller’s environment, such as some of the machine registers, are saved on the stack
- The newly called function then allocates room on the stack for its variables
Heap
- Heap is the segment where dynamic memory allocation usually takes place
- The Heap area is managed by malloc, realloc, and free
- The Heap area is shared by all shared libraries and dynamically loaded modules in a process
Constants
- There are two simple ways in C to define constants:
- Using #define preprocessor
- Using const keyword