Intro
Risks
- Service and data unavailability
- Data leaks (client list, industrial secret, business plans, e-mail...)
- Bank fraud, credit cards stolen ...
Consequences
- Companies: losing money (stock market value)
- Services (water, electricity ...)
Vulnerabilities
- Vulnerabilities in physical systems
- Vulnerabilities in infrastructures as networking systems
- Vulnerabilities in Operative Systems
- Vulnerabilities in Software
CIA Triad
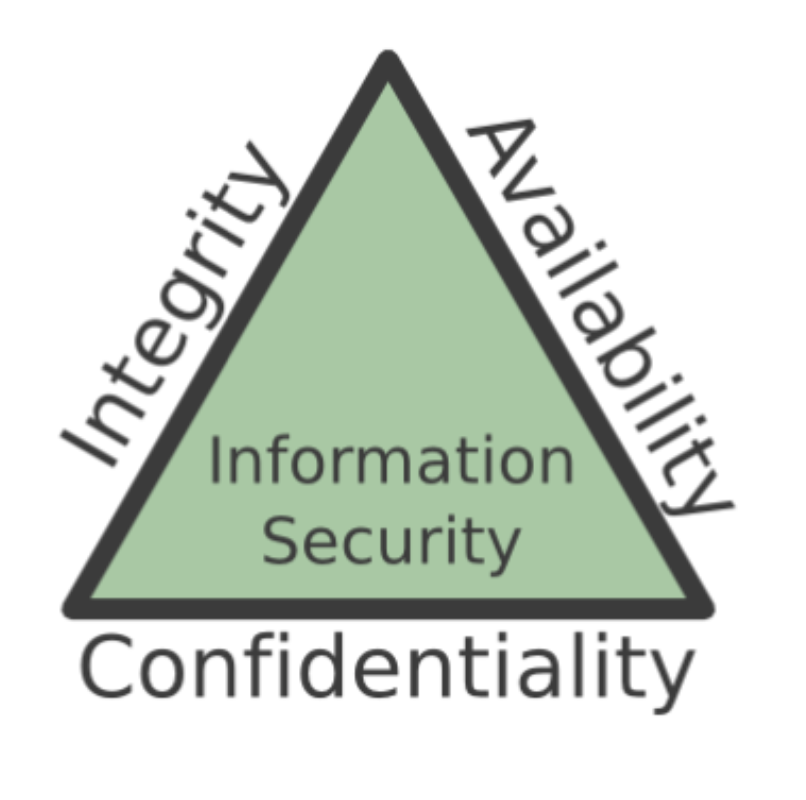
Simple Example
#include <stdio.h>
#include <string.h>
int main (int args, char** argv) {
char buffer[500];
strcpy(buffer,argv[1]);
return 0;
}
- this program copy argv in a buffer
- this program can do everything
Severe Vulnerability
- Why? Because this program has a severe buffer overflow vulnerability
- Buffer Overflow is a situation
- wherewecanaccidentallyoverflowabuffer meant to manipulate data and overwrite arbitrary memory addresses
- Overwritinglatermemoryaddresseswecancausemanykindsofproblems, including executing arbitrary code
- Wecanexploitthismaliciouslytoperformanattack,e.g.byoverwritingthe original program’s code with our own code
Vulnerability, Threats and Risk
Vulnerability
- weakness in the system
- Internal factor
Threat
- condition that can cause harm
- External factor
Risk
- Likelihood of the realisation of a threat
- Vulnerability without a threat = no risk
- hreat without a vulnerability = no risk
CVE/CVSS/CWE
- In 1999 MITRE (no-profit company) introduced an official uniform catalogue of vulnerabilities
- CVE – Common Vulnerabilities and Exposures
- CVSS – Common Vulnerability Scoring System
- CWE – Common Weakness Enumeration
Low-level Programming Languages & C
C memory layout
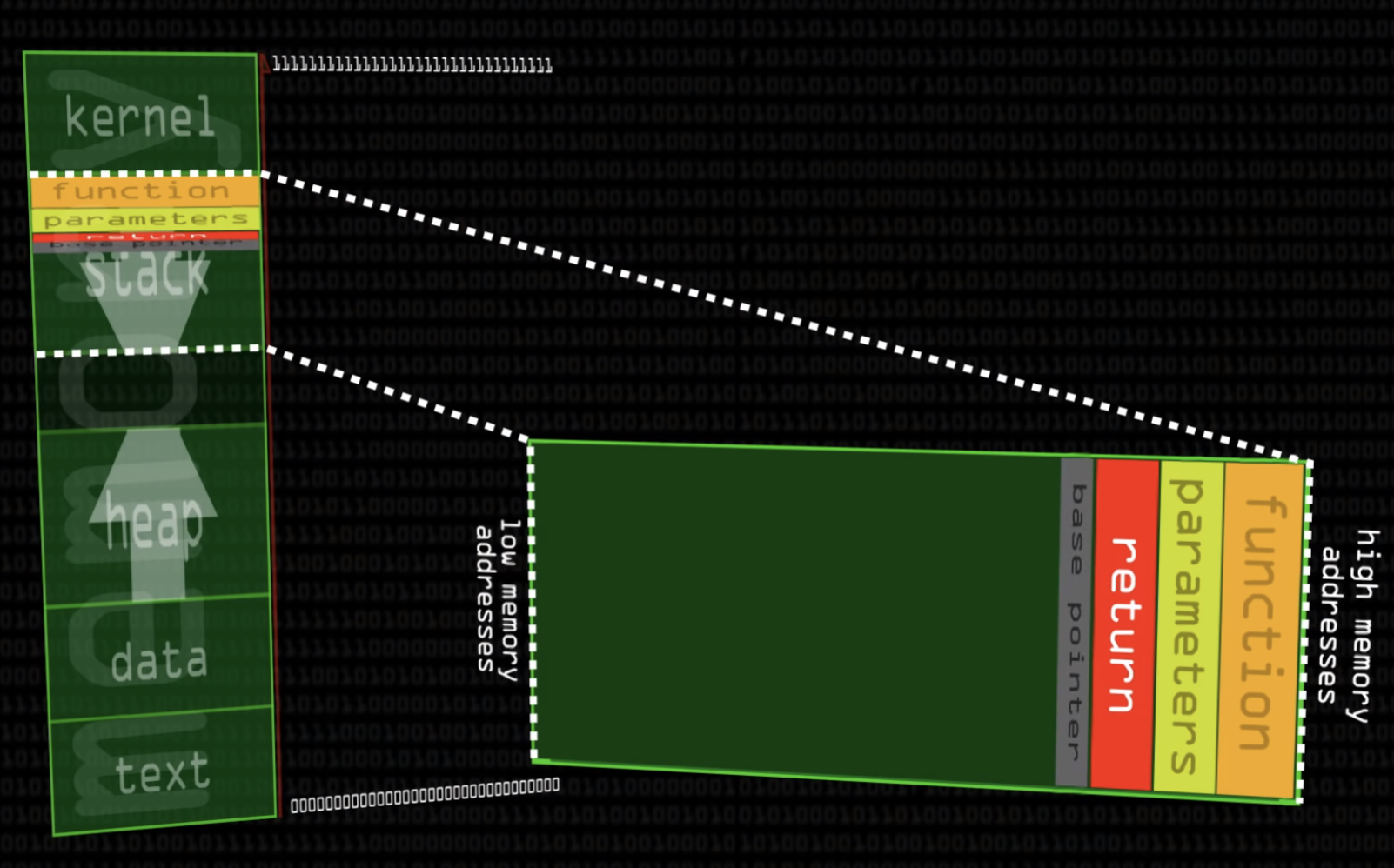
#include <stdio.h>
#include <string.h>
int main (int args, char** argv) {
char buffer[500];
strcpy(buffer,argv[1]);
return 0;
}
- Use this code for an example
- What happens if put 508 bytes
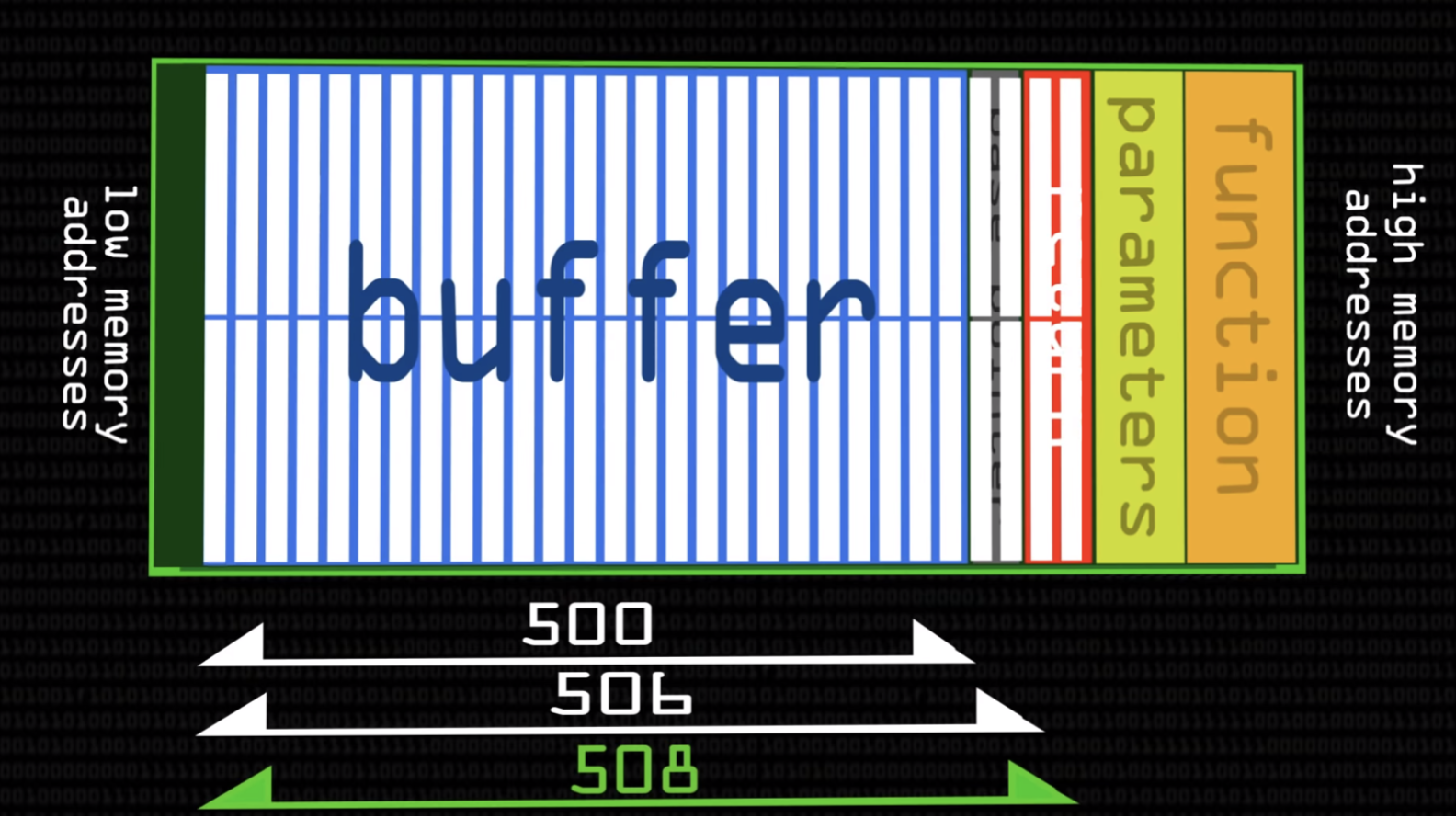
On re-entry, the code will try to jump back to a possibly illegal address, causing a so-called “Segmentation Fault” and a crash, a total system failure.
we can be more evil
- executeanarbitrarycodelike A shell, a malware, etc.
- Which of course gives me a lot of control over the machine, and sends all the CIA properties packing...
Low-level v High-level languages
- High-level are more “programmer” friendly
- Low-level languages are difficult to understand, read and code
- Machine Code is impossible to read
- C is the language of all software libraries, so the foundation of our current software stacks
- Assembly can be used for analysis of decompiled code