Intro to React
What is React
- React is a JavaScript library for building user interfaces created by Facebook.
- React allows us to create reusable UI components.
- A component takes in parameters, called props (short for “properties”), and returns a hierarchy of views to display via the render method.
- React allows us to write JavaScript and HTML in the same file (JSX)
Understanding the Virtual DOM
- React uses a virtual DOM to handle page rendering.
- In SPA where the view is highly interactive, directly manipulate a web page via the HTML DOM can cause performance issues.
- Virtual DOM is an in-memory representation of the real DOM.
- Unlike the HTML DOM, the virtual DOM is much easier to manipulate.
- Handling numerous operations in milliseconds without affecting page performance.
- React periodically compares the virtual DOM and the HTML DOM.
What is JSX?
• JavaScript and HTML in the same file
• JSX stands for JavaScript XML
• JSX allows us to write HTML tags within JavaScript functions
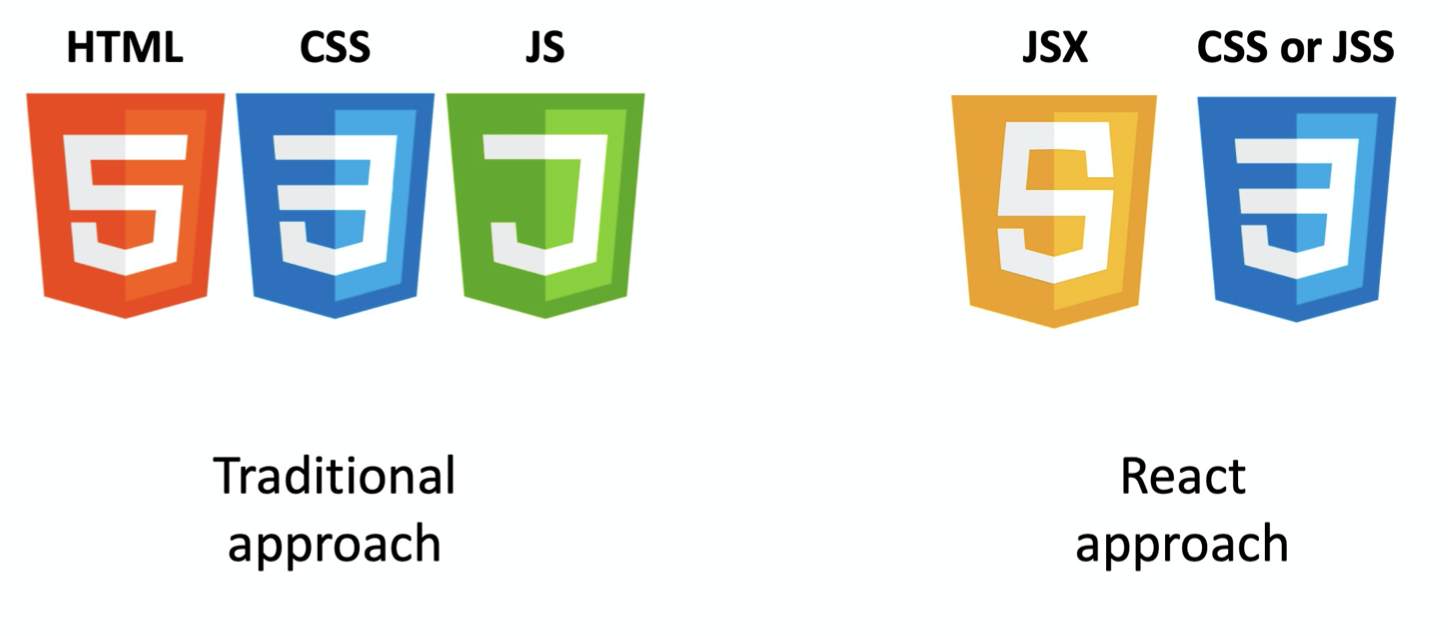
Common tasks in front-end development
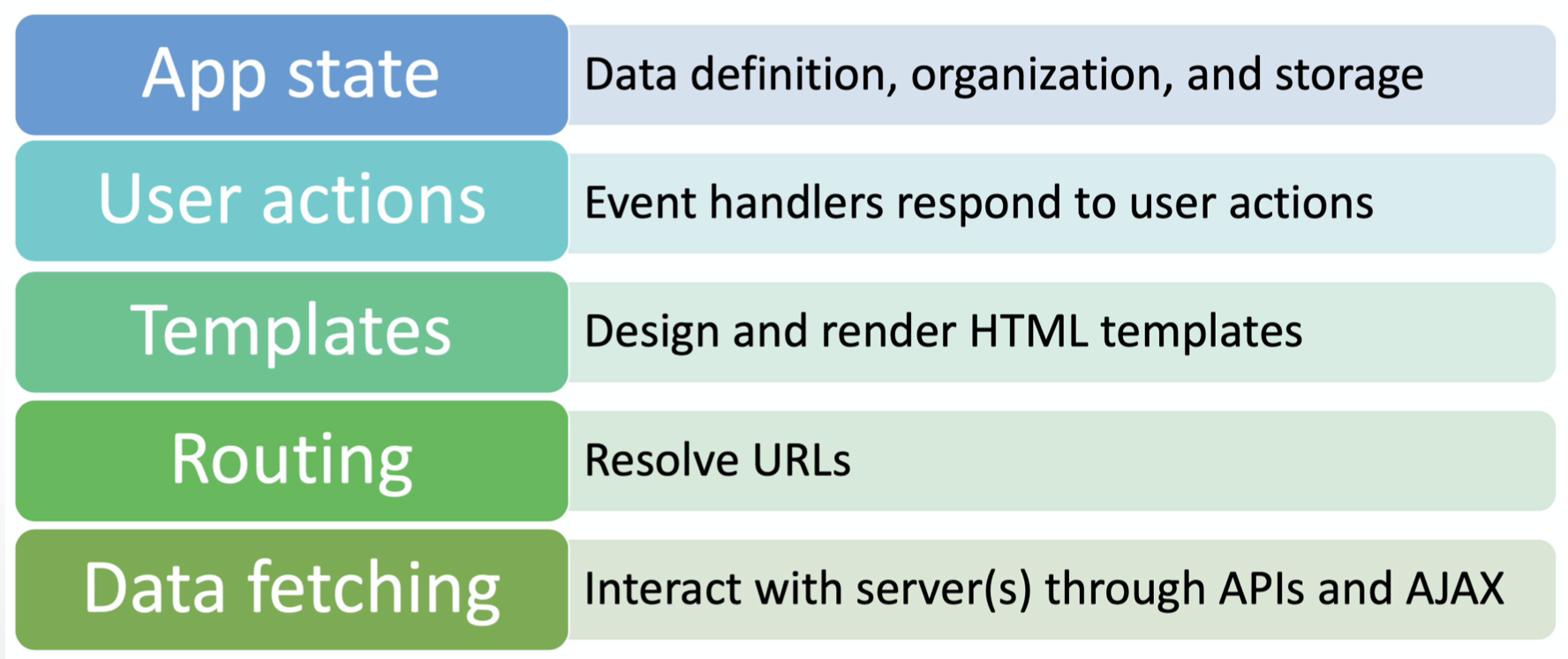
Anatomy of a React app
- index.js is the file first loaded (is used to generate index.html)
- Index.js willrenderthe component called App
- If you replace App with any other component, those will be rendered
- Uppercase tags are assumed to be React JSX
React Components
A component is combination of
- Template using HTML
- User Interactivity using JS
- Applying Styles using CSS
Components come in two types, Class components and Function components
Create a Class Component
- When creating a React component, the component's name must start with an upper case letter.
- The component has to include the extends React.Component statement, this statement creates an inheritance to React.Component, and gives your component access to React.Component's functions.
- The component also requires a render() method, this method returns HTML.
Create a Function Component
A Function component also returns HTML, and behaves pretty much the same way as a Class component.
Component Constructor
- You can have a constructor() function in your component, this method will be called when the component gets initiated.
- The constructor is where you initiate the component's properties.
- In React, component properties should be kept in an object called state.
- You also include the super() statement, which executes the parent component's constructor function, and allow your component to have access to all the functions of the parent component (React.Component).
React Props
- Another way of handling component properties is by using props.
- Props are like function arguments, and you send them into the component as attributes.
- To send props into a component, use the same syntax as HTML attributes.
- Props are also allow you pass data from one component to another, as parameters.
React Class Component State
- React components has a built-in state object.
- The state object is where you store property values that belongs to the component.
- When the state object changes, the component re-renders.
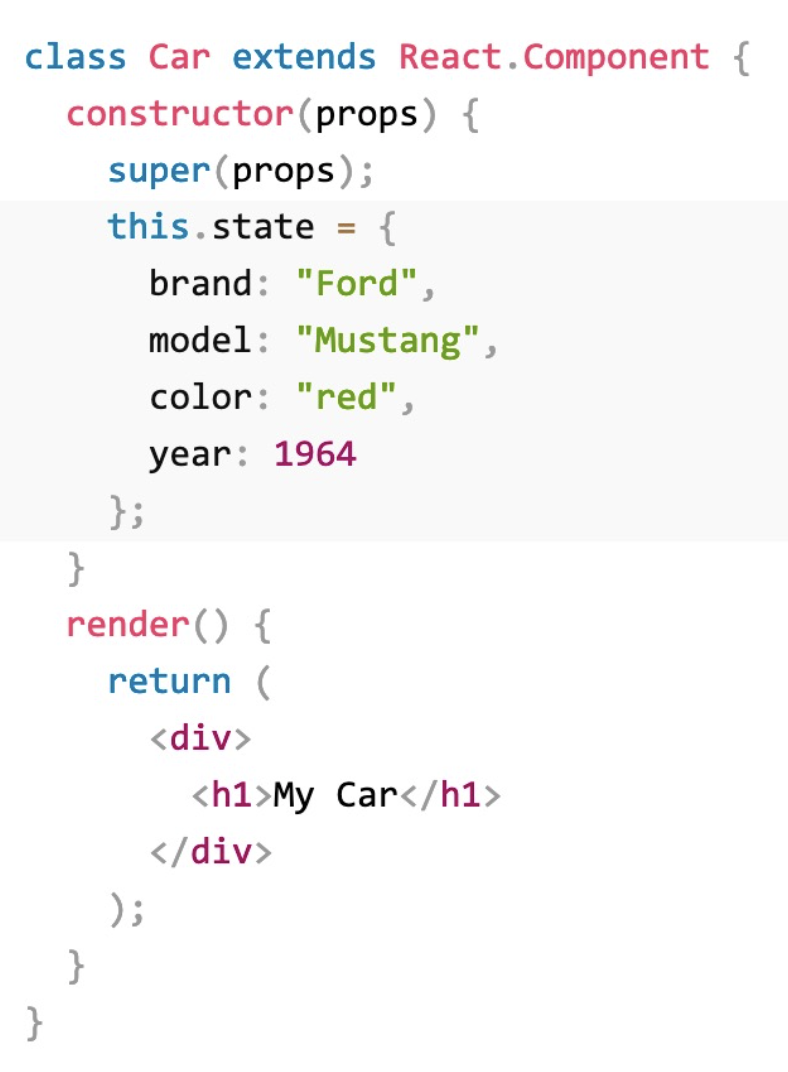
Class-based vs Function Components in React
- Class components have a state and provide access to lifecycle methods like componentDidMount.
- stateless functional components are pure functions that do not have a state or lifecycle methods. They take in props and return html.
- With the addition of Hooks, Function components are now almost equivalent to Class components.
Hooks
What are React Hooks?
- a feature lets you use state and life-cycle methods without writing a class component.
- React Hooks allow us to take Stateless Functional Components and apply state and lifecycle methods to them.
- Hooks can only be called inside React function components.
Q&A
Q1 Common Web Framework and their Functionalities
- URL routing
- Input form managing and validation
- HTML, XML, JSON, and other product setups with a templating engine
- Database connection configuration and resolute data manipulation through an object-relational mapper (ORM)
- Web security against Cross-site request forgery (CSRF), SQL Injection, Cross-site Scripting (XSS) and other frequent malicious attacks
- Session managment and retrieval
Q2 Front-end & Back-end Frameworks Development & Languages
Back-end (Server-side) Languages
Almost any programming language (C#, Java, Objective-C,PHP,Ruby,Python etc.) can be used to build server-side applications.
Back-end Frameworks
- ASP.NET(C#)
- Flask, Django(Python)
- Express(JS, Node.JS)
- Laravel(PHP)
Front-end Development & Languages
- HTML — Hypertext Mark-up Language: It is used to design thethe structure of web pages.
- CSS — Cascading Style Sheet: Allows you to apply styles to web pages independent of the HTML that makes up each web page.
- JS — JavaScript: A scripting language used to make the site interactive for the user.
Q3 Dynamic Websites/Applications
A dynamic site is one that can generate and return content based on the specific request URL and data (rather than always returning the same hard-coded file for a particular URL).
- Using the example of a product site, the server would store product "data" in a database rather than individual HTML files.
- When receiving an HTTP GET Request for a product, the server determines the product ID, fetches the data from the database, and then constructs the HTML page for the response by inserting the data into an HTML template.
- Using a database allows the product information to be stored efficiently in an easily extensible, modifiable, and searchable way.
- Using HTML templates makes it very easy to change the HTML structure, because this only needs to be done in one place, in a single template, and not across potentially thousands of static pages.
Q4 Single Page VS Traditional Web Apps
web site that interacts with the user by dynamically rewriting the current page rather than loading entire new pages from a server.
- making the application behave more like a desktop application.
- SPAs serve a single page of html and use in page controls to operate dynamically.
- Traditional web apps need to send many http requests, and get many html pages from the server. However Spas only need to initate the request get one html page, and use ajax to transfer data between front and back.
Q5 HTML structural Elements vs CSS Styles
- The browser has its own default styling for each heading level.
- However, these are easily modified and customized via CSS.
Q6 Different style of using CSS in conjunction with your HTLM files
Q7 Different concepts of functional programming in JavaScript such Immediately invoked function, Call-back, Promise, and so on
Immediately invoked function
- An immediately invoked function expression (IIFE) is a design pattern that produces a lexical scope using function scoping.
- IIFE can be used to avoid variable hoisting from within blocks or to prevent us from polluting the global scope.
Call-back
- A callback is a function to be executed after another function has been executed
- Callbacks are the most common way to write and handle asynchronous logic in JavaScript programs
- In JavaScript, functions are objects. Functions can therefore take functions as arguments and can be returned by other functions. Functions that perform this operation are called higher-order functions. Any function that is passed as an argument is called a callback function.
Promise
- It serves the same function as callbacks but has a nicer syntax and makes it easier to handle errors.
- It can be used to write better asynchronous code.
- a promise represents the result of an *asynchronous operation
Q8 JSX Syntax and its pro and Con
JSX Syntax
- Using JSX we can pass around HTML structures, or React elements as if they were standard JavaScript values.
- JavaScript and HTML in the same file
- JSX stands for JavaScript XML
- JSX allows us to write HTML tags within JavaScript functions
Pro
- The program structure is easier to visualize
- JSX is more flexible
Con
- jsx is not a template, and it's hard to understand
- Code of jsx does not look neat
Q9 Stateful and Stateless Components, different phases of React class component’s lifecycle
Stateful and Stateless components
- React components can further be categorized into stateful and stateless components.
- A stateless component’s work is simply to display data that it receives from its parent React component.
- If it receives any events or inputs, it can simply pass these up to its parent to handle.
- A stateful component, on the other hand, is responsible for maintaining some kind of application state
- This might involve data being fetched from an external source, or keeping track of whether a user is logged in or not.
- A stateful component can respond to events and inputs to update its state.
Lifecycle of Components
- Each component in React has a lifecycle which you can monitor and manipulate during its three main phases.
- The three phases are: Mounting, Updating, and Unmounting
React Component Lifecycle Diagram
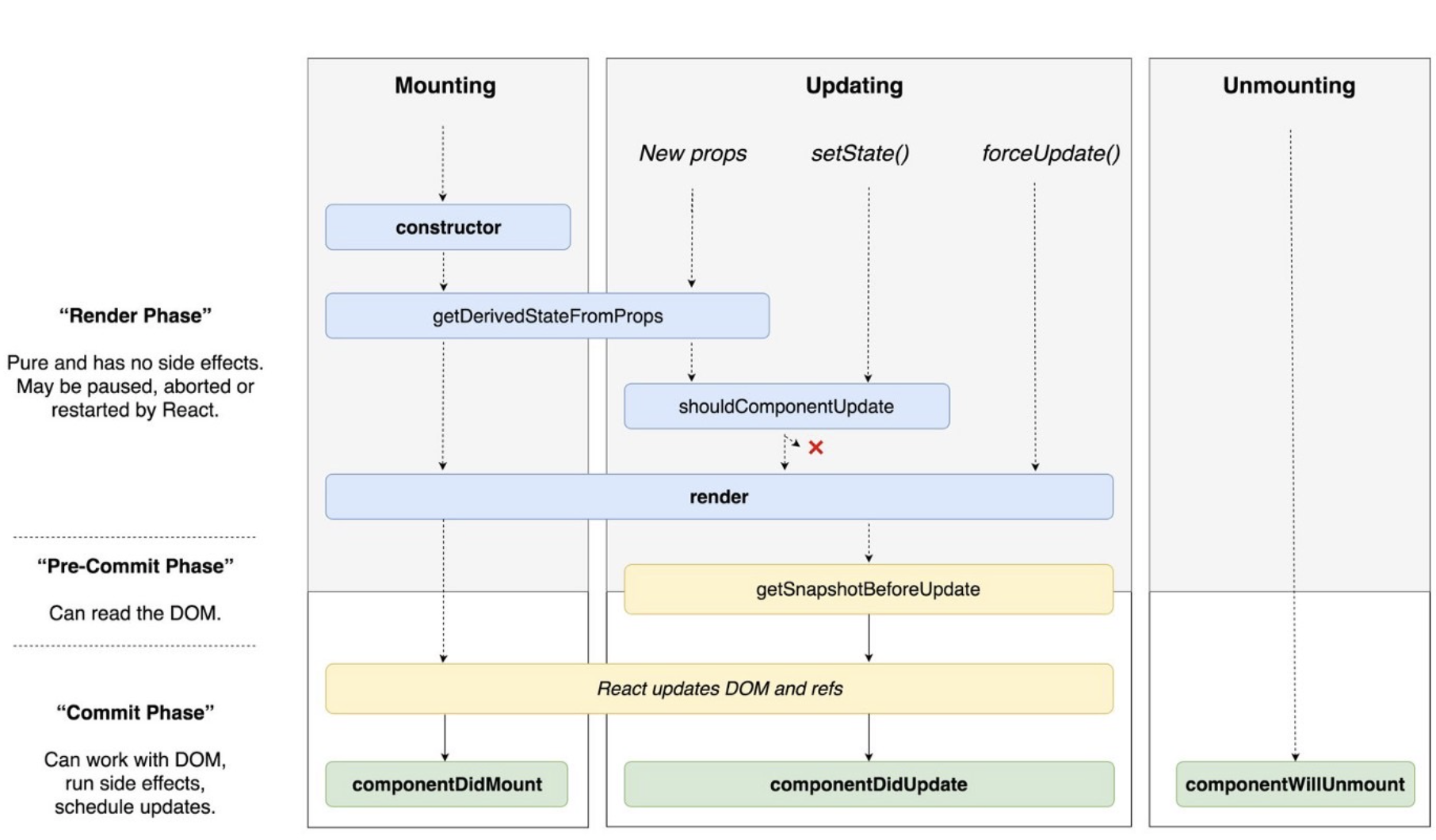
Lifecycle of Components – Mounting
- Mounting : means putting elements into the DOM.
- React has four built-in methods that gets called, in this order, when mounting a component:
- constructor()
- getDerivedStateFromProps()
- render()
- componentDidMount()
- React has four built-in methods that gets called, in this order, when mounting a component:
- The render() method is required and will always be called, the others are optional and will be called if you define them.
- The constructor() method is called before anything else, when the component is initiated, and it is the natural place to set up the initial state and other initial values.
- The getDerivedStateFromProps() method is called right before rendering the element(s) in the DOM.
- The componentDidMount() method is called after the component is rendered.
Lifecycle of Components – Updating
A component is updated whenever there is a change in the component's state or props.
Lifecycle of Components – Unmounting
The next phase in the lifecycle is when a component is removed from the DOM, or unmounting as React likes to call it.
Q10 Class-based vs Function Components in React
Class-based vs Function Components
- Class-based components will implement a render function, which returns some JSX (React’s extension of Regular JavaScript, used to create React elements),
- Whereas function components will return JSX directly.
Q11 Limitations of the Class-style Components
Limitations of the Class-style Components
- did not provide good code reuse and component structure capabilities.
- harder to share logic between class components without using design patterns and reder props and higher-order components.
- class components are harder to understand, more complex, and require more time and effort to maintain.
- need for a simpler implementation:Hooks
Q12 What are React Hooks?, Understanding of different kinds of Hooks
What are React Hooks?
- a feature lets you use state and life-cycle methods without writing a class component.
- React Hooks allow us to take Stateless Functional Components and apply state and lifecycle methods to them.
- Hooks can only be called inside React function components.
七大 Hooks 都有哪些
useState
状态useEffect
钩子,还有它的兄弟useLayoutEffect
useContext
上下文useReducer
代替 ReduxuseMemo
缓存,还有它的小弟useCallback
useRef
引用自定义 Hook
混合