Pointers
- A pointer is a variable that contains the address of a variable
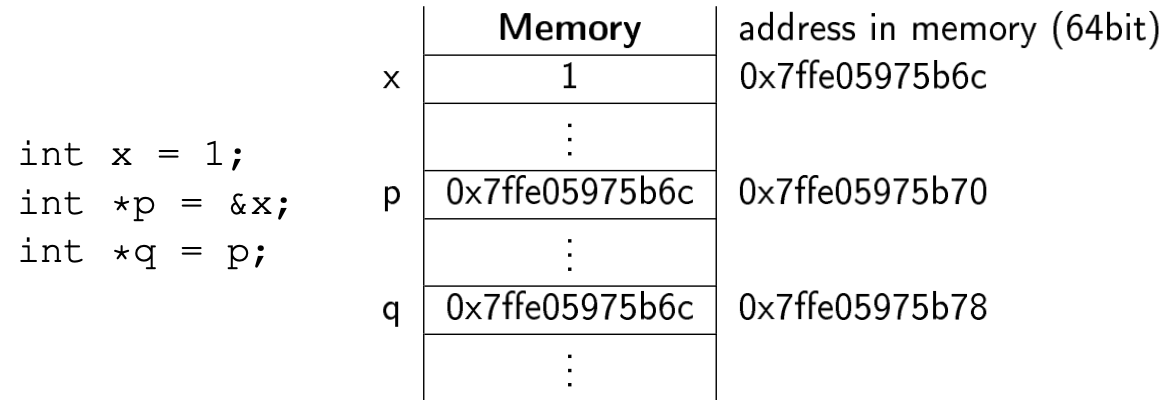
int x=1, y=2, z[10];
/∗ip is a pointer to int∗/
int ∗ip;
/∗ip now points to x ∗/
ip = &x;
/∗y is now 1∗/
y = ∗ip;
/∗x is now0∗/
∗ip = 0;
/∗ x = x + 1 ∗/
∗ip = ∗ip + 1;
/∗ ip now points to z[0] ∗/
ip = &z[0];
/∗ y = z[0] + 1 ∗/
y = ∗ip + 1;
++∗ip;
(∗ip)++;
int∗iq = ip;
Pointers to Pointers
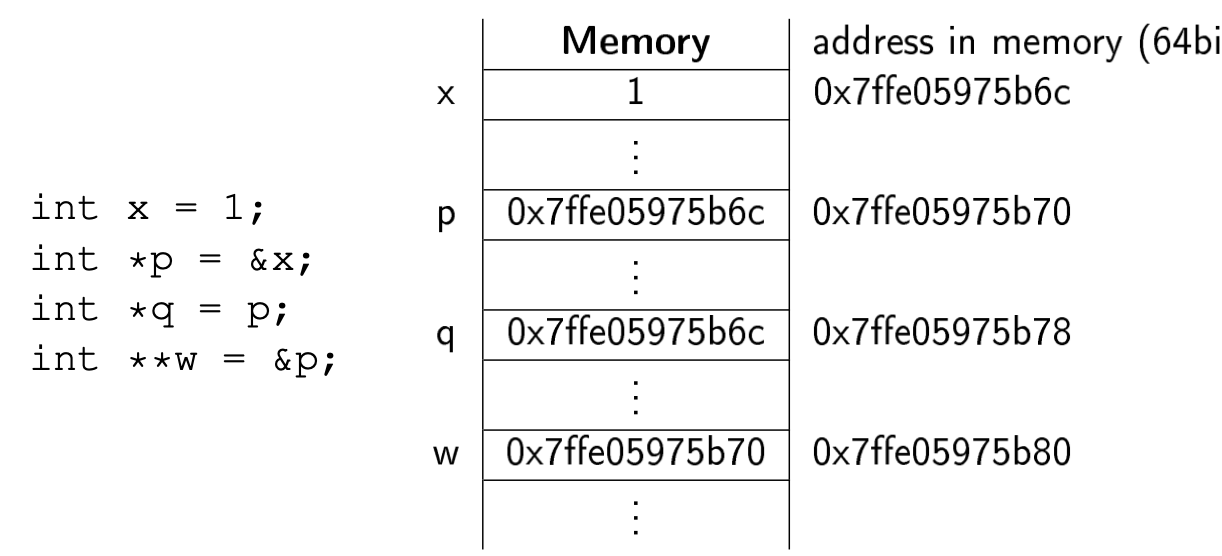
Pointer arithmetic
- the variable pointer can be incremented
- the formula for computing the address of pa + i where pa has type T*:
addr(pa + i) = addr(pa) + [sizeof(T) * i]
- i is scaled according to the size of the objects pa points to, which is determined by the declaration of p
- If an int is four bytes, for example, then i will be scaled by four
Null Pointers
int *ptr = NULL; /* ptr = 0*/
- use address 0 because that memory is reserved by the OS, so access to address 0 is not permitted.
- can use if statement to check null pointer
Return pointer from functions
C allows you to return a pointer from function. It is not good to return the address of a local variable to outside of the function.
/∗ if compilation failed , use gcc −ansi or gcc −std=c89 ∗/
#include <stdio .h>
#include <time.h>
int ∗ getRandom(){
static int r[10];
int i;
srand((unsigned)time(NULL)); /∗ set the seed ∗/
for (i = 0; i < 10; ++i)
r[i] = rand();
return r;
}
int main () {
int ∗p; int i;
p = getRandom();
for ( i=0; i<10; i++)
printf("∗(p+[%d]) :%d\n", i, ∗(p+i) );
return 0;
}
Pointers and Arrays
- In C, there is a strong relationship between pointers and arrays
int a[10];
int *pa;
pa = &a[0]; /* pa = a*/
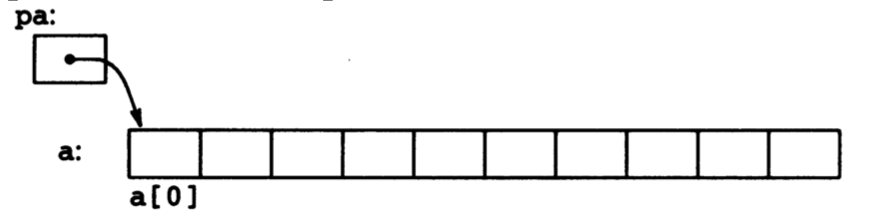
int x = *pa; /* same as x = a[0] */
- the variable pointer can be incremented, but the array name cannot, because it is a constant pointer.
- pa + i point i elements after pa, and pa - i points i elements before
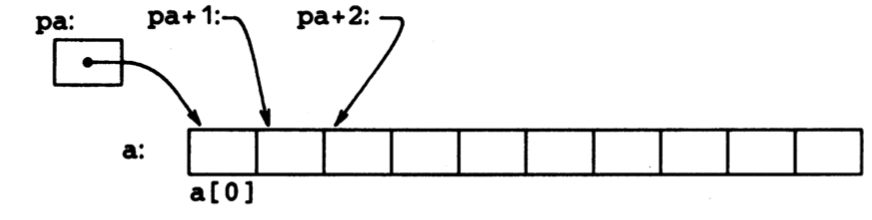
The name of an array is a synonym for the location of the initial element
a | pa | &a[0] |
---|---|---|
*a | *pa | a[0] |
a+1 | pa+1 | &a[1] |
a+i | pa+i | &a[i] |
*(a+1) | *(pa+1) | a[1] |
*(a+i) | *(pa+i) | a[i] |
Pointers to Functions
- in C, it is possible to define pointers to functions
int (*func)(int, int);
- like pointer to variables, function pointers can be assigned, placed in arrays, passed to functions, returned by functions, ...
#include <stdio .h>
int max (int, int);
int main ()
{
int (∗func)(int, int);
func = max; /∗ or func = &max ∗/
printf("%d\n", func(3, 4));
return 0;
}
int max(int a, int b)
{
return a>b ? a : b;
}
Pointers to constants and constant pointers
- A constant pointer is a pointer that cannot change the address its holding
int * const ptr;
- A pointer to constant is a pointer through which we cannot change the value of the variable it points to.
const int * ptr;
- we could have both in one definition
const int * const ptr;
Strings
- string in C is actually a one-dimensional array of characters which is terminated by a null character '\0'
- a null-terminated string contains the character that comprise the string followed by a null
char greeting[6] = {'H','e','l','l','o','\0'};
/* same as */
char greeting[] = "Hello";
- The C compiler automatically places the '\0' at the end of the string

- there is a difference between these definitions:
char amessage[] = "now is the time";
char *pmessage = "now is the time";
- Individual characters within the array may be changed but amessage will always refer to the same storage
- pmessage points to a string constant and may be modied to point elsewhere, but the result is undened if you try to modify the string contents
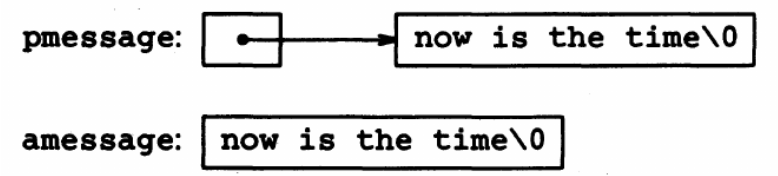
String IO
- reading string:
- using scanf(): scanf("%s", str);
- reading it character by character using getchar()
- using gets() but it is unsafe and dangerous, never use it. It continues reading until ‘\n’ or EOF
- printing strings:
- using printf(): printf("%s\n", str);
- printing it character by character using putchar()
- using puts(str) to print the entire string
Structures
- structure is a user dened data type that allows to combine data items of different kinds
- used to represent a record
- format of the struct statements:
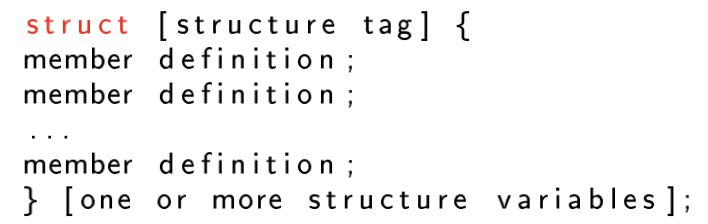
- to access member of a structure, use .
Structures as Function Arguments
#include <stdio .h>
#include <string .h>
struct Books { ... };
void printBook(struct Books book);
int main( ) {
struct
...
printBook ( book1 ) ;
return 0;
}
void printBook(struct Books book){
printf( "title : %s\n", book. title );
printf( "author : %s\n", book.author);
printf( "book_id : %d\n", book.book_id);
}
- can pass a structure to a function as a pointer
- use -> to access the members
Bit Fields
- Bit Fields allow the packing of data in a structure. This is especially useful when memory or data storage is at a premium
- Typical examples include:
- Packing several objects into a machine word. e.g. 1 bit ags can be compacted
- Reading external (non-standard) le formats, e.g., 9-bit integers
Unions
- union is a special data type available in C that enables you to store different data types in the same memory location
- define a union with many members, but only one member can contain a value at any given time
union Data {
int i;
float f;
char str[20];
} data;
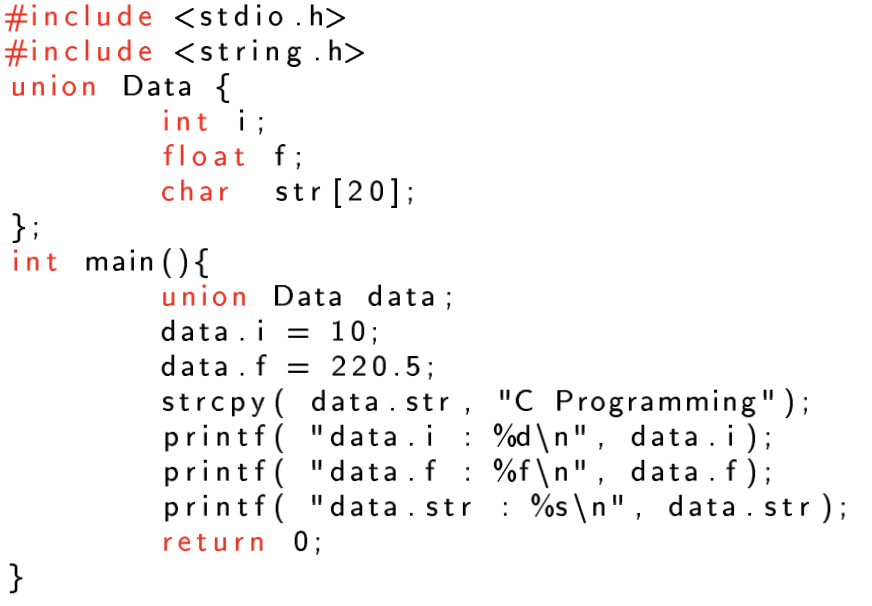